In modern software development, managing dependencies is critical to ensure security, maintainability, and compliance. Outdated or vulnerable dependencies can lead to security breaches, downtime, or compliance violations. Automating this process saves time, reduces human error, and ensures a more secure software lifecycle.
Whether you're focused on security or any other domain, this example provides a scalable framework for implementing multi-agent workflows. By the end of this guide, you'll not only have a working agent but also insights into adapting it for your specific requirements.
What are Multi-agents?
Multi-agent systems allow multiple specialized agents to work together autonomously to solve a problem. These agents perform distinct roles, such as data collection, analysis, decision-making, and reporting. By using LangGraph, we can easily define these tasks as nodes in a workflow and manage the communication between them.
Each agent performs a focused task and passes results to the next in the workflow. This modularity ensures:
- Scalability: you can add, modify or replace agents as needed.
- Flexibility: conditional logic allows for skipping unnecessary steps.
- Maintainability: focused responsibilities make debugging and updates easier.
Why LangGraph?
LangGraph is a library designed to orchestrate and manage Multi-agent workflows. It provides a framework for connecting independent tasks (agents) in a logical sequence, enabling seamless collaboration between them. Whether you’re building a simple pipeline or a complex AI-powered system, LangGraph.js makes it easier to manage dependencies, state transitions, and modular workflows.
At its core, LangGraph.js:
- Defines nodes: each task or agent is represented as a node in a directed graph.
- Manages edges: connections between nodes determine the workflow's sequence and logic.
- Handles state: passes data between nodes while maintaining a clear and predictable state structure.
By abstracting the complexity of Multi-agent coordination, LangGraph.js allows developers to focus on implementing agent logic rather than workflow mechanics.
Enhanced workflow with LangGraph
As mentioned we are going to build a dependency scanner Multi-agent with the following graph structure:

Breaking down the nodes and edges
- Dependency scanner: this node reads a package.json file and extracts the list of dependencies and their versions. Executes conditionally: if the dependency list is empty, the workflow ends; otherwise, it proceeds to the next node.
- Vulnerability analyzer: uses Gemini (gemini-1.5-flash) to analyze each dependency for known vulnerabilities.
- Secure alternatives: suggests secure and up-to-date alternatives for dependencies with vulnerabilities by using OpenAI (GPT-4o-mini).
- Report generator: consolidates all data from previous states into a readable report summarizing vulnerabilities and secure alternatives.
Code hands-on
Step 1: Setting up the project
First, you need to install Node.js on your computer if you haven't already done so. You can download and install Node.js from the official website: https://nodejs.org/en/download/
Then we Initialize the project and start by creating a new Node.js project with TypeScript and installing the basic dependencies, using the following commands:
mkdir dependency-scanner-agent
cd dependency-scanner-agent
npm init -y
npm install @langchain/langgraph @langchain/core @langchain/google-genai @langchain/openai dotenv
npm install -D typescript ts-node @types/node
npx tsc --init
Then change your tsconfig.json to this:
{
"compilerOptions": {
"target": "ES2020",
"module": "CommonJS",
"strict": true,
"esModuleInterop": true,
"outDir": "./dist",
"rootDir": "./src"
},
"include": ["src"],
"exclude": ["node_modules"]
}
The folder structure at the end will be something like this:
dependency-scanner-agent/
├── src/
│ ├── index.ts >> defines the graphs nodes and edges
│ ├── agent.ts >> defines the graphs state and routing
│ └── nodes/
│ ├── Agents folder
├── README.md
├── package.json
├── tsconfig.json
Step 2: Get your LLM’s Gemini/OpenAI API Credentials
Our agents use OpenAI and Gemini to produce results, so you will need to set up API credentials for those models. To do this you can follow the steps we already shared in past articles for OpenAI and Gemini.
Once you have your API key, create a .env file in your project directory and place these variables:
OPENAI_API_KEY=your_openai_api_key
GEMINI_API_KEY=your_gemini_api_key
Step 3: Implementing the agents
Now let’s implement the agents that will handle dependencies scanning, vulnerability analysis, and report generation.
1. Agent state and routing
Create a new TypeScript file called agent.ts in your project root directory, and add the following code:
CODE: https://gist.github.com/pablanco/b539c82e415f763b9c966d267a894f44.js?file=gistfile1.ts
2. Dependency scanner
Create a new TypeScript file called dependencyScanner.ts in your project nodes directory, and add the following code:
CODE: https://gist.github.com/pablanco/efb41cda4e3e9e3ad3f5c3f211edd355.js?file=gistfile1.ts
3. Vulnerability analyzer
Create a new TypeScript file called vulnerabilityAnalyzer.ts in your project nodes directory, and add the following code:
CODE: https://gist.github.com/pablanco/9c5d2963e0f9826c6ca9b57f3723e383.js?file=gistfile1.ts
4. Secure alternatives
Create a new TypeScript file called secureAlternatives.ts in your project nodes directory, and add the following code:
CODE: https://gist.github.com/pablanco/5de4a4d603dcec0c1470983de8ad2fa6.js?file=gistfile1.ts
5. reportGenerator
Create a new TypeScript file called reportGenerator.ts in your project nodes directory, and add the following code:
CODE: https://gist.github.com/pablanco/ec74c81c412ce1f12aabfe9a4340ea8b.js?file=gistfile1.ts
Step 4: Define the main workflow
All the previous agents need to be orchestrated somehow, to do that agents are connected in a workflow. We define the workflow in a new TypeScript file called index.ts in your project root directory, and add the following code:
CODE: https://gist.github.com/pablanco/5bc5821163f9f056f313b807c984eb3d.js?file=gistfile1.ts
Step 5: Run the Agent
Change your package.json to have a new start command by adding an entry to the “scripts”:
"scripts": {
....
"start": "ts-node src/index.ts"
}
Now start the application using the following commands:
npm run start
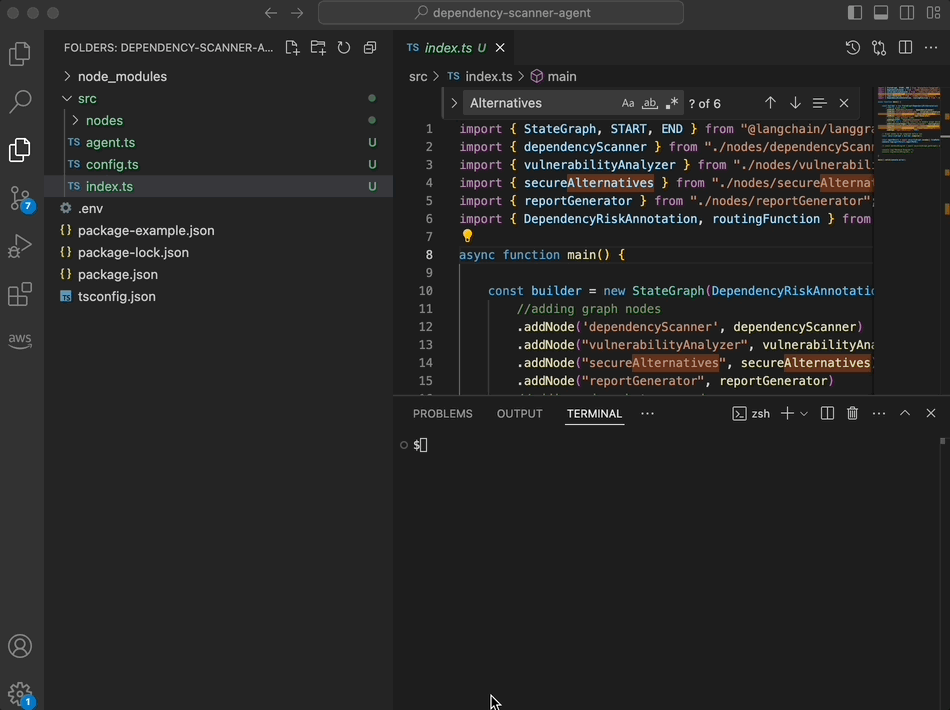
When you run the app, agents will check your package.json file in order to check dependencies, after the agent ends you can check a new report created in dependency_security_report.md. That's it! You have now successfully built your Agent with Node.js and LangGraph.
Code repository example
You can get the working code example from this repository: https://github.com/rootstrap/langgraph-nodejs
Summary
This example demonstrates how multi-agent workflows with LangGraph.js simplify the automation of complex processes, ensuring scalability and adaptability. By integrating powerful tools like OpenAI and Gemini, developers can tackle real-world challenges with ease.
By combining LangGraph.js, Gemini, and OpenAI, this project highlights how Multi-agent systems can automate critical workflows, reducing human error and improving efficiency.
Some future work ideas
- Add support for other dependency managers like pip for Python: extend the tool to handle Python (requirements.txt) or Ruby (Gemfile) dependencies.
- Integrate into CI/CD pipelines: automate execution as part of the build process to ensure dependency security throughout development.
- Integrate notifications: send alerts via Slack or email for critical vulnerabilities.